Array:C Programming Language - Part 6
Array
In previous programs we have worked with two variables. We may have to write programs that work on more than one variable. We use arrays in programming to work with more than one variable of the same type.
For example, the first five prime numbers are 2, 3, 5, 7, 11. Now we will use these five numbers in our program. If we want to use normal variables, then we have to declare five variables. But using the array we can use these five prime number programs using a variable.
Arke can think of a box like this, which can contain many small boxes, see the table below.
1 2 3 4 5 ... n
Now, to keep us in the top five prime numbers in our program, we will take a box containing 5 boxes. And take a prime number in each box. Below the following:
2 3 5 7 11
Instead of 5, we can keep a number of prime numbers in just one array. When we say to the Arke, what is there in the number one, it will return us two. Similarly, if I say no, then it will return 11.
Declare array:
To use arrays, first to declare an array. Arrays are declared just like other normal variables. Such as
Data_type array_name [size]
The data type tells us what kind of data we will keep in the array. If you keep the intestine, then int, if you put the character then you have to say char. Character can not be placed in integer arrays. Floating point can not be placed in the integer array again.
The key is to give a name to the array. Next size. We will keep some alimants in the array by size. For example, to keep five prime numbers, we can write an array like the following:
Int prime [5];
Here we have created an array of 5 named Elements. That means we'll keep 5 integer numbers in it. In this way we can create arrays for other data type as follows:
Float gpa [100];
Char name [30];
Array Initialize
When we create variables, such as a value can be assigned, we can tell the elimants while creating the array.
Int prime [5] = {2, 3, 5, 7, 11};
Called array initialization. When we created the array, we also told its illimant. Comma is to be used for the item from one element to another. And the values are written in the second bracket.
Above we have initialized an array in one line. If we want, we can initialize differently, as follows:
1
2
3
4
5
6
Int prime [5];
Prime [0] = 2;
Prime [1] = 3;
Prime [2] = 5;
Prime [3] = 7;
Prime [4] = 11;
Floating point array
Float gpa [4] = {3.5, 3.8, 3.9, 2.9};
Array declares its elimants while not saying the size of the array will not be a problem. Such as
Float gpa [] = {3.5, 3.8, 3.9, 2.9};
Access Array:
Array access to the array to find out the elements. Indexing array starts from 0. See the below array
Int prime [5] = {2, 3, 5, 7, 11};
The first element of this array is 0 in the index. The second element or the 1th index contains 3. Thus, fourth element or 3th index contains 11.
If we write: prime [0], it will return us two. If I write prime [1] it will return 3. When the prime [3] is written, it will return 11. See the following program
1
2
3
4
5
6
7
8
9
10
#include <stdio.h>
Int main ()
{
Int prime [5] = {2, 3, 5, 7, 11};
Printf ("% d", prime [0]);
Return 0;
}
In the above program, we just printed an array of arrays. You can now write 1, 2, 3, instead of the prime [0] written in the printf function. You will see the contents of one index once printed.
Before that we learned the loop. If we want to access all elements of an array, we can use the loop. Such as:
1
2
3
4
5
6
7
8
9
10
11
#include <stdio.h>
Int main ()
{
Int i;
Int prime [5] = {2, 3, 5, 7, 11};
For (i = 0; i <5; i ++)
Printf ("% d \ n", prime [i]);
Return 0;
}
Here if we have a hundred variables in our array, we can easily access it. Just replace i <5 instead of i <100.
Well we now think of a program that will take 6 numbers from the user and add it later and the result will show us. If we had to take 6 variables before then, then we had to add them and then sum would have to be shown.
Now we can easily do this thing with just one variable
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
#include <stdio.h>
Int main ()
{
Int number [6];
Int i, result = 0;
For (i = 0; i <6; i ++) {
Printf ("Enter% d no number: \ n", i + 1);
Scanf ("% d", & number [i]);
Result + = Number [i];
}
Printf ("result is:% d", result);
Return 0;
}
If we want, we can print any number of user inputs
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
#include <stdio.h>
Int main ()
{
Int number [6];
Int i, result = 0;
For (i = 0; i <6; i ++) {
Printf ("Enter% d no number: \ n", i + 1);
Scanf ("% d", & number [i]);
Result + = Number [i];
}
Printf ("Result is:% d \ n", result);
For (i = 0; i <6; i ++) {
Printf ("array element% d is:% d \ n", i, number [i]);
}
Return 0;
}
2D array
We have discussed the array so long that it was the One Dimensional Array. Arrays can be multi-dimensional. Such as 2D, 3D etc. Two Dimensional arrays are like below.
The array is declared as follows
Data_type array_name [i] [j];
There can be any number of values of i and j here. The size of the array will be i * j That means you can keep i * j illimant in the array. Now if i is 5, j is 6, then there are 30 arrays in that array. See the picture below:
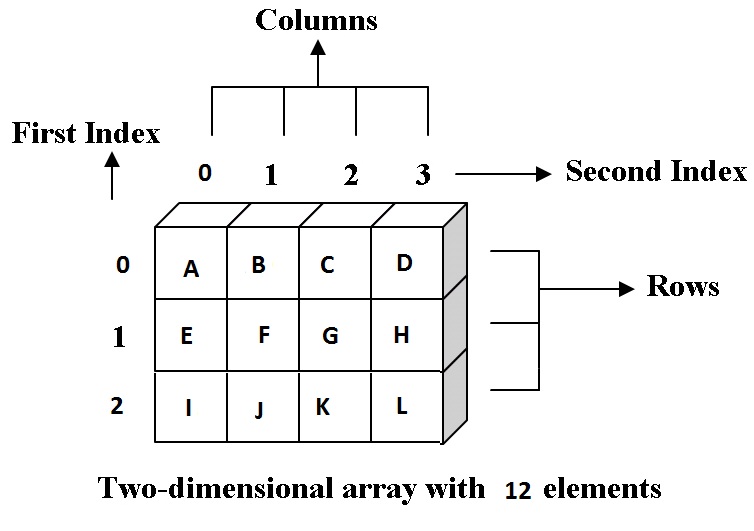
This is a two dimensional character array whose content is 12 Let's say Array's name is Character and it should be written in Character [3] [4] The first is row number, second is the column number. That is, there are 3 rows and 4 columns, the total number is 12.
The first element is the above Character called Two Dimensional Array
To get the first element, we need to write Character [0] [0] and it is A
To get the second element, we need to write Character [0] [1] and it is B
To get the third element, we need to write Character [0] [2] and it is C
To get the fourth element, we need to write Character [0] [3] and it is D.
To get the fifth element, we need to write Character [1] [0] and it is E.
To get the sixth element, we need to write Character [1] [1] and it is F
To get the seventh element, we need to write Character [1] [2] and it is G
In this way we can get the next element.
To keep something, follow the same steps.
Let's put a character in the first index, for that character [0] [0]
Already said Array Index starts from zero.
To place a Character in the second index, you must type Character [0] [1]
The rest should be kept in this way.
Think more easily if the above example sounds difficult. We have an array called int num [2] [2]. In this we can keep the maximum 4 elements. As we have an integer array
Int num [2] [2] = {1,2,3,4}
The computer will keep it as follows:
1 2
3 4
1
2
3
4
Int [0] [0] = 1;
Int [0] [1] = 2
Int [1] [0] = 3;
Int [1] [1] = 4;
Now if we have an int [0] [1] let's see, we will see 2 there. Which we can write in this way:
1
2
3
4
Int num [2] [2] = {
{1,2},
{3,4}
}
If we take an array named int num [3] [3] then we can have the maximum 9 elements. Below the following:
Int num [3] [3] = {1,2,3,4,5,6,7,8,9}
The program will keep it as follows:
1 2 3
4 5 6
7 8 9
That is to say
1
2
3
4
5
6
7
8
9
10
11
Int [0] [0] = 1;
Int [0] [1] = 2;
Int [0] [2] = 3;
Int [1] [0] = 4;
Int [1] [1] = 5;
Int [1] [2] = 6 '
Int [2] [0] = 7;
Int [2] [1] = 8;
Int [2] [2] = 9;
Which we can initialize in this way too
1
2
3
4
5
6
Int num [3] [3] = {
{1,2,3},
{4,5,6}
{7,8,9}
}
In the program, we can easily use 2d arrays using two for loops. See the following program:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
#include <stdio.h>
Int main ()
{
Int i, j;
Int num [2] [2] = {10,20,30,40};
For (i = 0; i <2; i ++)
{
For (j = 0; j <2; j ++)
{
Printf ("value of num [% d] [% d]:% d \ n", i, j, num [i] [j]);
}
}
}
If you run that you will get the following output:
1
2
3
4
Value of num [0] [0]: 10
Value of num [0] [1]: 20
Value of num [1] [0]: 30
Value of num [1] [1]: 40
See the following program:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
#include <stdio.h>
Int main ()
{
Int i, j;
Int num [2] [2] = {
{10,20},
{30,40}
};
For (i = 0; i <2; i ++)
{
For (j = 0; j <2; j ++)
{
Printf ("value of num [% d] [% d]:% d \ n", i, j, num [i] [j]);
}
}
}
Here in the other way the array is initialized. If you run, you get the same output.
In the above program we worked with a static 2D array. Now we can use input from the user.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
#include <stdio.h>
Int main ()
{
Int i, j;
Int num [2] [2];
// reading value
For (i = 0; i <2; i ++)
{
For (j = 0; j <2; j ++)
{
Printf ("Enter value of num [% d] [% d]:", i, j);
Scanf ("% d", & num [i] [j]);
}
}
// printing value
For (i = 0; i <2; i ++)
{
For (j = 0; j <2; j ++)
{
Printf ("You entered value of num [% d] [% d]:% d \ n", i, j, num [i] [j]);
}
}
Return 0;
}
Here we have an array of 2 * 2 size. If you want, you can work with any size array.
If we do not give value at the time of initializing the array, then it will be filled with null or zero. Look at the following arrow intentions:
1
2
3
4
5
Int num [3] [3] = {
{1,2},
{4,5,6}
{7,8}
};
We've got an array of 3 * 3 size. But I did not assign value to all. In whatever position we have not entered a value, we will get zero in those positions. You can run the following program:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
#include <stdio.h>
Int main ()
{
Int i, j;
Int num [3] [3] = {
{1,2},
{4,5,6}
{7,8}
};
For (i = 0; i <3; i ++)
{
For (j = 0; j <3; j ++)
{
Printf ("value of num [% d] [% d]:% d \ n", i, j, num [i] [j]);
}
}
Return 0;
}
<Strong> Passing an array in the function: </ strong>
<Strong> </ strong>
<Strong> </ strong>
If we want, we can pass an array as an argument to a function. See the following program
#include <stdio.h>
Int getSum (int arr []) {
Int sum = 0;
Int i;
For (i = 0; i <5; ++ i) {
Sum + = arr [i];
}
Return sum;
}
Int main () {
Int balance [5] = {25, 2, 3, 17, 50};
Printf ("Sum is:% d", getSum (balance));
Return 0;
}
Here we have written a function named getSum. Which has an integer array as a parameter. And when we call the function we passed an array.
No comments